Managing credentials and environment variables within Xcode and XCTest
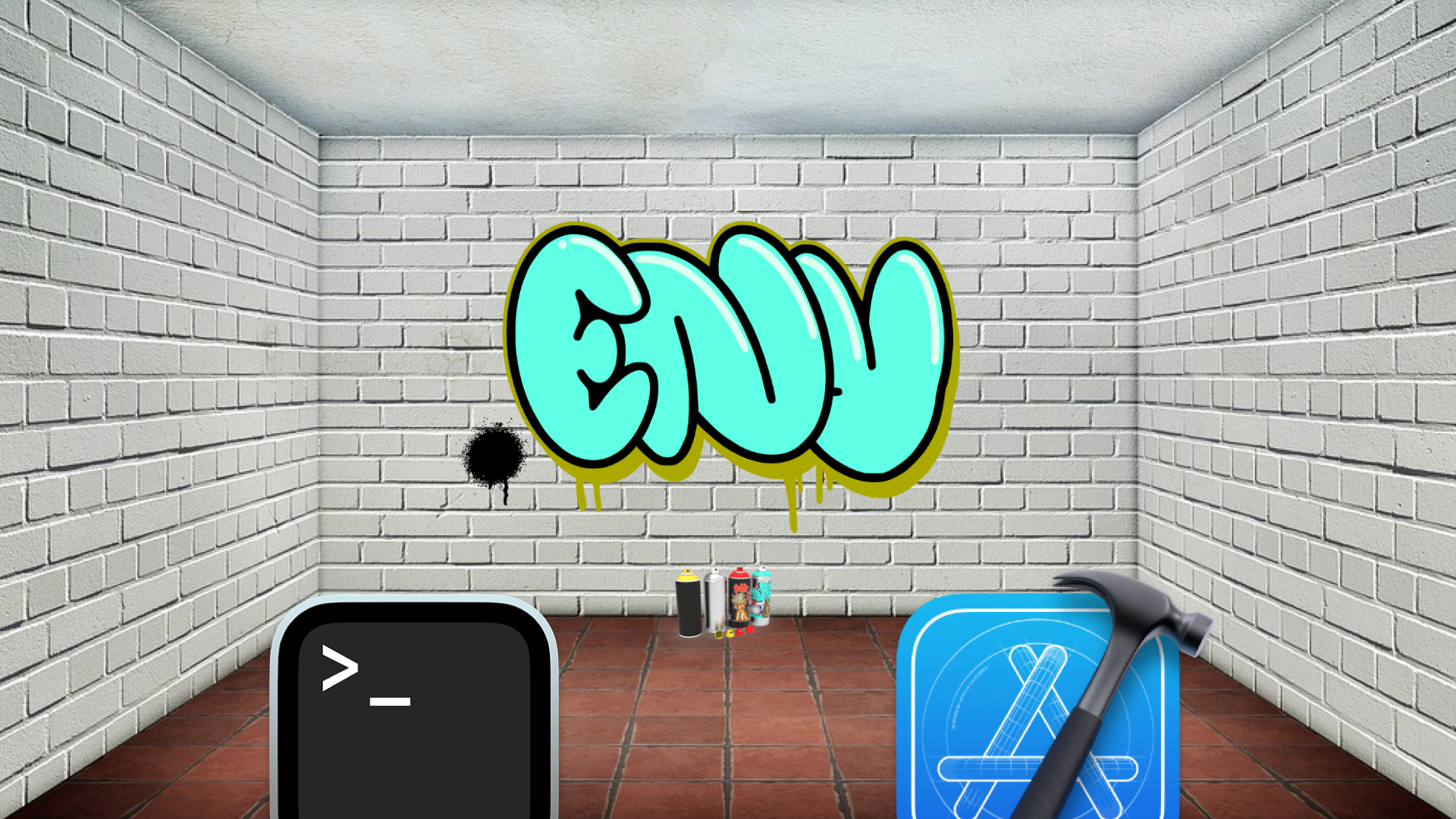
Credentials, what can be easier, right? Just get them from .env file or whatever and you’re good to go. Well, not so fast… not on iOS.
To be honest, it was a bit challenging to make it work from Xcode and from the Terminal at the same time. Passing credentials through the command-line only is not a problem at all, but as soon as you want to run these tests from Xcode it takes a ridiculous turn.
So, if you happen to be dealing with credentials in native tests on iOS, I’m afraid you’re limited to the following options:
- Hard-code credentials in the test code
- Pass credentials through a scheme or an xctestplan
Let’s pretend that the first option is not an option at all and jump straight to the second one.
Precondition
Create an environment variable in your xctestplan:
- Open the
.xctestplan
file - Click on the
Configurations
tab Arguments
section →Env Vars
row →➕
button- Add a new variable with the name
AWESOME_TOKEN
and the value$(AWESOME_TOKEN)
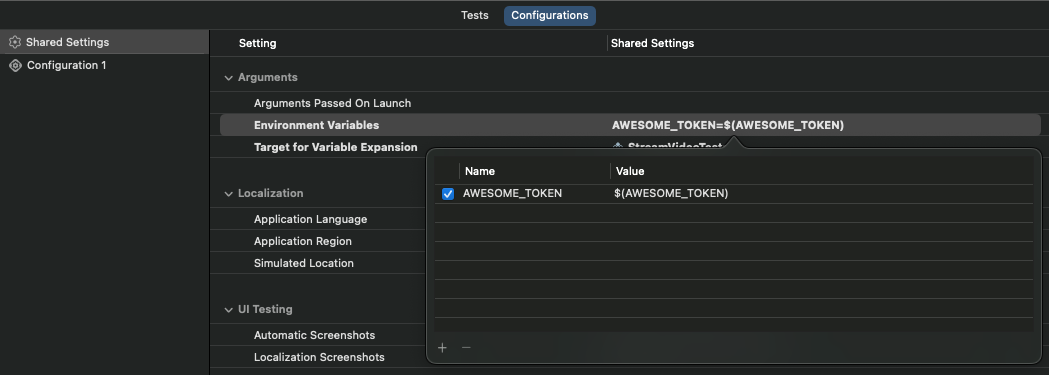
Create a sample test that prints the value of our secret token:
import XCTest
class SampleTestCase: XCTestCase {
func testToken() {
let awesomeToken = ProcessInfo.processInfo.environment["AWESOME_TOKEN"]!
print(awesomeToken)
XCTAssertFalse(awesomeToken.isEmpty)
}
}
How to manage it
in Xcode
- Create
env.xcconfig
file in your project- File → New → File… → Configuration Settings File
- Type the name of the file:
env.xcconfig
- Choose the location of the file
- Choose the target with your tests
- Attach
env.xcconfig
to the tests’ target- Click on your project name in the Project Navigator
- Open
Info
tab - Expand the
Debug
configuration in theConfigurations
section - Add
env.xcconfig
file to the app target for UI tests or to the test target for Unit tests
-
Add the
AWESOME_TOKEN
variable to theenv.xcconfig
fileAWESOME_TOKEN = 1234567890
- Add
env.xcconfig
file to.gitignore
! - Run the test 🤌
PS: you might want to share this file with your team so that they can run the tests as well.
on CI
- Create a secret variable in your CI (e.g.: GitHub Actions)
-
Create an empty
env.xcconfig
file on the fly so thatxcodebuild
command doesn’t failtouch env.xcconfig
-
Run the test 🤌
xcodebuild test \ -project "Sample.xcodeproj" \ -scheme "Sample" \ -destination "platform=iOS Simulator,name=iPhone 14" \ AWESOME_TOKEN=${{ secrets.AWESOME_TOKEN }} | xcpretty